PowerINSPECT Automation
Context
this document applies to :
- PowerINSPECT 2001 and later
Gripe no 000
Summary
PowerINSPECT 2.0 has support for Automation using Visual Basic. This allows
you to write Visual Basic applications that programmatically control
PowerINSPECT to automate repetitive tasks, for example.
Description
Project References
In Visual Basic use Project- References to refer to PowerINSPECT as shown.
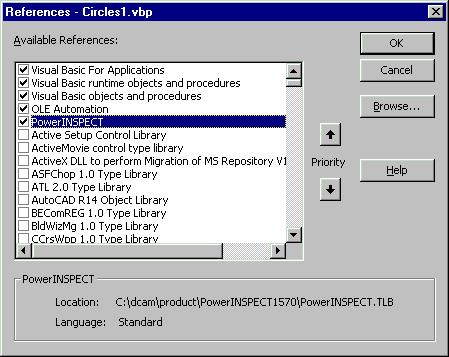
Selecting the active PowerINSPECT document
Use the code fragment shown below.
' Create the PowerINSPECT server object
Dim objPWIApplication As PowerINSPECT.Application
Set objPWIApplication = New PowerINSPECT.Application
' Get PowerINSPECT's active document
Dim objDocument As PowerINSPECT.Document
Set objDocument = objPWIApplication.ActiveDocument
' Check that there is an active document open
If (doc Is Nothing) Then
MsgBox "No Active Document!"
Exit Sub
End If
Declaring and using PowerINSPECT Groups
In this code fragment the variable objGeometricGroup refers to a Geometric Group.
' Add a geometric group to the definition level of the document's sequence tree
Dim objGeometricGroup As PowerINSPECT.ISequenceGroup
Set objGeometricGroup = objDocument.SequenceItems.AddGroup(pwi_grp_GeometricGroup)
Declaring and using PowerINSPECT Sequence Items
In this code fragment a Plane is created and then used as the reference plane for the
subsequent 3 circles. The variables objPlane and objCircle are defined as a PowerINSPECT
Geometric Sequence Items.
' Add a plane to the geometric group
Dim objPlane As PowerINSPECT.IGeometricItem
Set objPlane = objGeometricGroup.SequenceItems.AddItem(pwi_ent_Plane_Probed_)
objPlane.Name = "Plane for Circles"
' Add circles to the geometric group, using the plane as the reference plane
Dim objCircle As PowerINSPECT.IGeometricItem
Dim i As Integer
For i = 1 To 3
Set objCircle = objGeometricGroup.SequenceItems.AddItem(pwi_ent_Feat_ProbedCircle_)
objCircle.Name = "Circle " & i
objCircle.Links(1) = objPlane.Features.Item(1)
Next i
Retrieving a particular Sequence Item
' Get "Circle 1" from the geometric group
Dim objSequenceItem as PowerINSPECT.ISequenceItem
Set objSequenceItem = objGeometricGroup.SequenceItems("Circle 1")
Extracting a measurement value
' Get the radius property object from the circle
Dim objRadiusProperty As PowerINSPECT.PropertyRadialDimension
Set objRadiusProperty = objCircle.Properties(2)
' Get the measured value for the master part
Dim dblRadius As Double
dblRadius = objRadiusProperty.Measured("Master part")
Circles Example
This example creates a plane and circles using the plane as the reference
plane. It then creates another geometric group containing lines that join the
circles together.
Private Sub cmdCreate_Click()
' Get the name the user has chosen for the elements
' we are going to create from my text box "txtName"
Dim strName As String
strName = txtName.Text
If (strName = "") Then
' The user has not entered a name
MsgBox "Please enter a name to use when creating the entities", vbExclamation
Exit Sub
End If
' Get the number of circles the user wants us to create from my text box "txtNumCircles"
On Error Resume Next
Dim intNumberOfCircles As Integer
intNumberOfCircles = txtNumCircles.Text
If (Err.Number <> 0) Then
' There was an error converting the text box content to a number
MsgBox "Please enter the number of circles you want to create", vbExclamation
Exit Sub
End If
On Error GoTo 0
' Create the PowerINSPECT server object
' NOTE: This will start PowerINSPECT if it is not already running
Dim objPWIApplication As PowerINSPECT.Application
Set objPWIApplication = New PowerINSPECT.Application
' If we started a new PowerINSPECT then it will start invisible - make it visible
objPWIApplication.Visible = True
' If we made PowerINSPECT visible then PowerINSPECT will now be the
' front Windows application - make us the front application again
Me.Show
' Get PowerINSPECT's active document (if it has one)
Dim objDocument As PowerINSPECT.Document
Set objDocument = objPWIApplication.ActiveDocument
If (objDocument Is Nothing) Then
' There is currently no active document
' - ask the user if they want to create one
Dim enmAnswer As VbMsgBoxResult
enmAnswer = MsgBox("PowerINSPECT needs a document open to continue. Do you want to create one?", vbQuestion + vbOKCancel)
If (enmAnswer <> vbOK) Then
' The user does not want to continue
Exit Sub
End If
' Create a new document
objPWIApplication.Documents.Add
' Check that we now have an active document
Set objDocument = objPWIApplication.ActiveDocument
If (objDocument Is Nothing) Then
MsgBox "Can not create a new document", vbCritical
Exit Sub
End If
End If
' Add a geometric group to the definition level of the document's sequence tree
Dim objCirclesGeometricGroup As PowerINSPECT.ISequenceGroup
Set objCirclesGeometricGroup = objDocument.SequenceItems.AddGroup(pwi_grp_GeometricGroup)
objCirclesGeometricGroup.Name = strName & " Circles"
' Add a plane to the geometric group
Dim objPlane As PowerINSPECT.IGeometricItem
Set objPlane = objCirclesGeometricGroup.SequenceItems.AddItem(pwi_ent_Plane_Probed_)
objPlane.Name = "Plane for Circles"
' Add the circles to the geometric group, using the plane as the reference plane
With objCirclesGeometricGroup.SequenceItems
Dim objGeometricItem As PowerINSPECT.IGeometricItem
Dim i As Integer
For i = 1 To intNumberOfCircles
Set objGeometricItem = .AddItem(pwi_ent_Feat_ProbedCircle_)
With objGeometricItem
.Name = "Circle " & i
.Links(1) = objPlane.Features.Item(1)
End With
Next i
End With
' Add a second geometric group to the definition level of the document's sequence tree
Dim objLinesGeometricGroup As PowerINSPECT.ISequenceGroup
Set objLinesGeometricGroup = objDocument.SequenceItems.AddGroup(pwi_grp_GeometricGroup)
objLinesGeometricGroup.Name = strName & " Lines"
' Add lines to the second geometric group that join all the circles in the first geometric group
With objLinesGeometricGroup.SequenceItems
' NOTE: We are starting from item 2 in the circles geometric
' group because item one will be the reference plane
For i = 2 To (objCirclesGeometricGroup.SequenceItems.Count - 1)
Set objGeometricItem = .AddItem(pwi_ent_Line_ThrowPoints_)
With objGeometricItem
.Name = "Line " & i
.Links(1) = objCirclesGeometricGroup.SequenceItems(i)
.Links(2) = objCirclesGeometricGroup.SequenceItems(i + 1)
End With
Next i
End With
End Sub
Another Example
This example function creates a new document and adds a geometric group to
it. It then adds a number spheres to the geometric group and sets their
properties.
Private Sub CreatePISpheres(ByVal intNumberOfSpheres As Integer, _
ByVal dblSphereDiameter As Double, _
ByVal dblSpherePositionX As Double, _
ByVal dblSpherePositionY As Double, _
ByVal dblSpherePositionZ As Double, _
ByVal dblSphericity As Double)
' Create the PowerINSPECT server object
' NOTE: This will start PowerINSPECT if it is not already running
Dim objPWIApplication As PowerINSPECT.Application
Set objPWIApplication = New PowerINSPECT.Application
' Ensure PowerINSPECT is visible to the user
objPWIApplication.Visible = True
' Add a new document to PowerINSPECT
Dim objDocument As PowerINSPECT.Document
Set objDocument = objPWIApplication.Documents.Add
' Add a geometric group to the definition level of the document's sequence tree
Dim objGeomtricGroup
Set objGeomtricGroup = objDocument.SequenceItems.AddGroup(pwi_grp_GeometricGroup)
objGeomtricGroup.Name = "Spheres"
' Add the sphere's to the geometric group
Dim objSphere As PowerINSPECT.IGeometricItem
Dim i As Integer
For i = 1 To intNumberOfSpheres
' Add the sphere
Set objSphere = objGeomtricGroup.SequenceItems.AddGeometricItem(pwi_ent_Feat_Sphere)
With objSphere
' Set the name of the sphere
.Name = "Sphere " & i
' Set the nominal diameter of the spheres
Dim objRadialDimensionProperty As PowerINSPECT.IPropertyRadialDimension
Set objRadialDimensionProperty = .Properties(1)
objRadialDimensionProperty.Nominal = dblSphereDiameter
objRadialDimensionProperty.LowerTol = -0.05
objRadialDimensionProperty.UpperTol = 0.05
' Set the nominal coordinates for the spheres
Dim objPointProperty As PowerINSPECT.IPropertyPoint3
Set objPointProperty = .Properties(2)
objPointProperty.SetNominals i * dblSpherePositionX, dblSpherePositionY, dblSpherePositionZ
objPointProperty.SetUpperTolerances 0.01, 0.01, 0.02
objPointProperty.SetLowerTolerances -0.01, -0.01, -0.02
' Set the nominal sphericity for the spheres
Dim objGeometricToleranceProperty As PowerINSPECT.IPropertyGeometricTolerance
Set objGeometricToleranceProperty = .Properties(3)
objGeometricToleranceProperty.MaxValue = dblSphericity
End With
Next
End Sub
Problem cause
N/A
Solution/Workaround
N/A